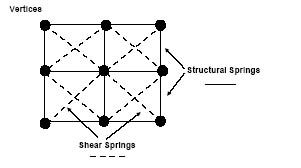
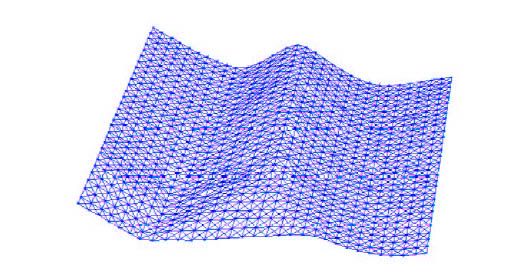
//Irregular mesh . . ... . . . ......//plus regluar rectangle. . . . . . . . . . ... . . . ......//plus lines . . . . . . . .| | | | | | | | | | | | | | | || | | . . ... . | | | . . ......//equals regular mesh . . . . . . . .//YAY!:-)
Quote: Original post by Steve132
Well, I seem to remember your post from before, and while I dont think that this would give you a mathematically PERFECT solution, it might give you one that is darn close. Here is what I would do to solve your problem:
You have your "kinda flattish" Irregular mesh like in your previous thread. IT is composed of a whole bunch of vertices kinda scattered around that make a kind of book shape. All of those together give a surface after they are connected by triangles: What I would do is this: take a flat big rectagle, that is made up of regular squares and is the approximate depth and width of the irregular shape. Put the vertices of the whole thing at a position above your irregular mesh, and then for each of the vertices of the regularlly meshed rectangle, (algorithmically, of course: by hand would suck a lot) Find the place where a vertical line through the vertex would intersect the irregular mesh. You may have to do some planar algebra because of the fact that your lines probably wont intersect the irregluar surface at a specific vertex. Then, the points of intersection should be a new, approximatly regular mesh.
Anyway, that is my idea at least. If my horrible ASCII art didnt illustrate the concept, At least I tried :-). Good Luck!
Quote: Original post by llvllatrix
Hi,
Your file contains both vertices and tesselation data (that is the realtion between the vertices). You should be able to construct an approximation of the mesh using this data alone (cast a ray vertically at a given x,z point of your regular mesh and have it collide with your irregular; the collision point gives you your y value). The result would be a mesh alligned with the coordinate axis and it would look like someone took a piece of cloth and hung it over your model (there would be overhang).
You might also be able to use the texture coordinates to determine the z value of the irregular mesh. The texture coordinates essentially describe how to map a plane to the model. Basically find the extremes of the texture coordinates (ie the lowest and highest) and sample starting from the lowest and moving towards the highest. At each sample, use interpolation between the vertices of the mesh and their relation to the texture coordinate to determine the x, y, and z of the point. The result would be a regular mesh with no overhang and would be alligned with the trends of your model. Be advised however that the texture coordinates that are in the obj file you provided are overly large (i imported the model into milkshape).
If you manually import the file into a model editor, create your own regular mesh, and then export the result you will still be left with the same information that you began with. That is you will still just get vertices and faces. If you intend to manipulate the mesh then you will still need to go through the process of recreating the regular mesh in order to establish the planar relationship between vertices.
Hope that helps and good luck,
- llvllatrix